Groovy Power Assert
When I googled for 'power assert', the first result found was the JavaScript library power assert. The second link contains an interview with the author of this library. He acknowledges the inspirations from Groovy ecosystem. Borrowing good ideas from another language/ ecosystem has had positive influence on many languages.
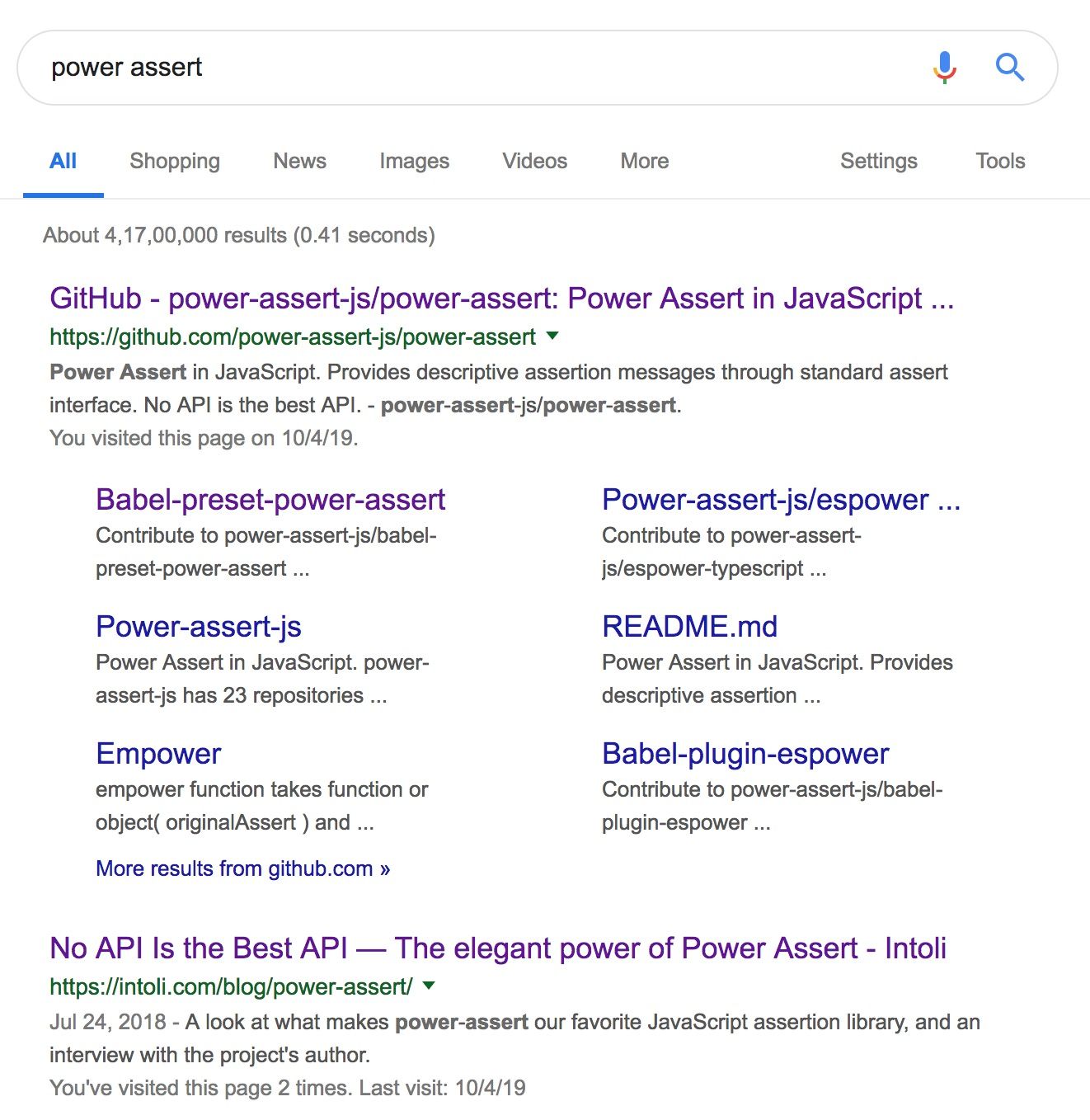
Let's see them in action, off course in Groovy!
def a = 10
def b = 4
assert a + b == 15
In the above example, we are asserting if the value of the expression a+b
is equal to 15. Since the assertion will fail, you will receive the following error.
Caught: Assertion failed:
assert a + b == 15
| | | |
10| 4 false
14
Assertion failed:
assert a + b == 15
| | | |
10| 4 false
14
at demo.PowerAssertion.run(PowerAssertion.groovy:5)
Notice how nicely the output shows what the expression evaluates to. With this a developer can quickly understand what is going on here and fix the problems. (Yes the stacktrace has the expression mentioned twice, which is an area for improvement!)
Let's take another example.
assert [1, 2, 3, 4]
.findAll { it % 2 == 0 }
.sum() == 5
Following is the error produced. I have omitted the duplicate message for ease of reading.
Assertion failed:
assert [1, 2, 3, 4] .findAll { it % 2 == 0 } .sum() == 5
| | |
[2, 4] 6 false
at demo.PowerAssertion.run(PowerAssertion.groovy:8)
Notice how the intermediate results are expressed, which makes it easy to spot the problems.
Spock is a test framework in Groovy ecosystem. Spock takes advantage of the power assert feature. In Spock assert is implied in 'expect' and 'then' blocks. Have a look at the following example.
package demo
import spock.lang.Specification
class SampleSpec extends Specification{
def "sample test"() {
given:
def a = 10
def b = 4
expect:
a + b == 15
}
}
Note how I did not have to mention 'assert' in the expect block. Here is the result of running the above specification.
Condition not satisfied:
a + b == 15
| | | |
10| 4 false
14
<Click to see difference>
at demo.SampleSpec.sample test(SampleSpec.groovy:13)